
In Magento 1.x there is a special function for getting access to directories – Mage::getBaseDir(). In Magento 2.x it has been removed, but a whole new class has been added to fulfill the same mission. Please welcome Magento\Framework\Filesystem\DirectoryList.
Let’s take a look at the main methods of the class:
File lib/internal/Magento/Framework/Filesystem/DirectoryList.php:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
---------------------------------------------------------------------------------- <?php namespace Magento\Framework\Filesystem; class DirectoryList { ... public function getRoot() { return $this->root; } public function getPath($code) { $this->assertCode($code); return $this->directories[$code][self::PATH]; } public function getUrlPath($code) { $this->assertCode($code); if (!isset($this->directories[$code][self::URL_PATH])) { return false; } return $this->directories[$code][self::URL_PATH]; } ... } |
One of the ways to use these functions is to declare this class in __constract:
1 2 3 4 5 6 7 8 9 10 11 12 |
protected $_dir; ... public function __construct( ... \Magento\Framework\Filesystem\DirectoryList $dir, ... ) { ... $this->_dir = $dir; ... } |
Now you can use these methods to do the following:
– Get root Magento directory:
1 |
$this->_dir->getRoot(); |
– Get any working Magento directory (for example ‘var’):
1 |
$this->_dir->getPath(‘var’); |
– Connect url to the working directory of Magento:
1 |
$this->_dir->getUrlPath(‘media’); // return relative url – 'pub/media' |
Here is the list of working directories available as arguments:
base, app, code, etc, lib_internal, var, cache, log, di, generation, i18n, session, media, static, pub, lib_web, tmp, sys_tmp, design, upload, view_preprocessed, html, setup.
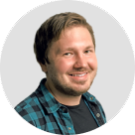