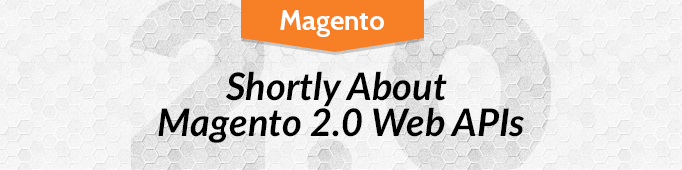
APIs* can be used for a wide variety of tasks, from creating shop applications to CRM*/ERP* integrations. It’s quite easy to start working with APIs. Let’s take a look at creating products and categories using token-based* authentification. To do this we need Composer and Magento 2.0.
Let’s create a simple API call example for importing product and category to the webstore database.
First of all lets create a separate user for API calls and limit it’s access to catalog operations. Roles and users management interface is located under System -> Permissions menu tab and is self-explanatory.
I’ll use the Guzzle library for sending API request. In order to install this tool via composer use instruction
:
1 2 3 4 5 |
{ "require": { "guzzlehttp/guzzle": "~6.0" } } |
Now we can start creating the application (in this post I tried to complicate the code).
First of all we need client class for processing our requests:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
<?php class Client { protected $_token; protected $_client; public function __construct($url, $username, $password) { $this->_client = new GuzzleHttp\Client(['base_uri' => $url]); try { $response = $this->_client->request('POST', '/rest/V1/integration/admin/token', [ 'json' => [ 'username' => $username, 'password' => $password ] ]); $this->_token = str_replace('"', '', $response->getBody()->getContents()); } catch (GuzzleHttp\Exception\ClientException $e) { echo $e->getRequest(); if ($e->hasResponse()) echo $e->getResponse(); } return $this; } public function getToken() { return $this->_token; } public function getClient() { return $this->_client; } } |
Client allows us to run token-based auth and initialize API session.
Now let’s try to make some API calls.
The first one is for adding a product:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<?php $username = 'apiUser';
$password = 'apiPassword'; $testProduct = array( 'sku' => "product-webapi", 'name' => "Product WebApi", 'price' => '19.95', 'weight' => '10', 'status' => '1', 'visibility' => '4', 'attribute_set_id' => '4', 'type_id' => 'simple', );
try {
$webapi = new Client('http://example.com/index.php', $username, $password); $response = $webapi->getClient()->request('POST', '/rest/V1/products', [ 'headers' => [ 'Authorization' => "Bearer " . $webapi->getToken() ], 'json' => [ 'product' => $testProduct ] ]); var_dump(json_decode($response->getBody())); } catch (GuzzleHttp\Exception\ClientException $e) { echo $e->getMessage(); }
|
In this example we created $testProduct array with required product information for import and send POST request to the appropriate URL.
As a response we should get a JSON object that contains the information about the newly created product.
And now we can make similar request for category creation
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<?php $testCategory = array( 'parent_id' => '2', 'name' => 'Category WebApi', 'is_active' => '1', 'include_in_menu' => '1', 'available_sort_by' => array('position', 'name'), 'custom_attributes' => array( array('attribute_code' => 'description', 'value' => 'Custom description'), array('attribute_code' => 'display_mode', 'value' => 'PRODUCTS'), array('attribute_code' => 'is_anchor', 'value' => '0'), ), );
try {
$webapi = new Client('http://example.com/index.php', $username, $password); $response = $webapi->getClient()->request('POST', '/rest/V1/categories', [ 'headers' => [ 'Authorization' => "Bearer " . $webapi->getToken() ], 'json' => [ 'category' => $testCategory ] ]); var_dump(json_decode($response->getBody())); } catch (GuzzleHttp\Exception\ClientException $e) { echo $e->getMessage(); }
|
After some modification and optimization this code can be used for importing products from a CSV or some remote source.
To find out more about the possibilities of Magento 2 Web APIs, I recommend you to check source texts in Magento 2.0 root: dev/tests/api-functional/testsuite
Good luck!
API – in computer programming, an application programming interface (API) is a set of routines, protocols, and tools for building software and applications.
CRM – customer relationship management (CRM) is an approach to managing a company’s interaction with current and future customers.
ERP – enterprise resource planning (ERP) is a category of business-management software—typically a suite of integrated applications—that an organization can use to collect, store, manage and interpret data from many business activities.
Token-based authentication is a process of getting a token (session identifier) using login and password in order to work with remote website resources during a defined timeframe (without entering login and password).

Hi Aaron,
thanks for your suggestion.
Nice article, I only have one suggestion:
You shouldn’t use str_replace to remove the quotes, because it’s a JSON string. json_decode will return the proper result and should be used uniformly when dealing with the M2 REST API.
Thank’s!