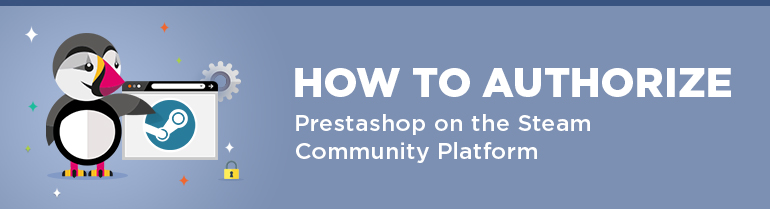
This article is dedicated to the authorization process for Prestashop on Steam, specifically, to the SteamCommunity platform.
Steam is a game and software digital distribution service.
If you are going to sell games, registration keys, skins or any other Steam products through Prestashop you will need to know user’s Steam ID.
So, let’s begin. First we create a new module SteamLogin in the file /modules/steamlogin/config.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 |
<?xml version="1.0" encoding="UTF-8" ?> <module> <name>steamlogin</name> <displayName><![CDATA[Steam login]]></displayName> <version><![CDATA[1.1.1]]></version> <description><![CDATA[Steam login]]></description> <author><![CDATA[belvg]]></author> <tab><![CDATA[front_office_features]]></tab> <confirmUninstall>Are you sure you want to uninstall?</confirmUninstall> <is_configurable>1</is_configurable> <need_instance>0</need_instance> <limited_countries></limited_countries> </module> |
Next, create the main module file /modules/steamlogin/steamlogin.php. Everything is pretty basic here so we will start from the constructor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<?php class SteamLogin extends Module { public function __construct() { $this->name = 'steamlogin'; $this->tab = 'front_office_features'; $this->version = '1.1.1'; $this->author = 'belvg'; $this->need_instance = 0; parent::__construct(); $this->displayName = $this->l('Steam login'); $this->description = $this->l('Steam login'); $this->confirmUninstall = $this->l('Are you sure you want to uninstall?'); } |
Then we will need a Steam Key to register in SteamCommunity and to generate an URL. Let’s make the following module set-up:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 |
public function displayForm() { $default_lang = (int)Configuration::get('PS_LANG_DEFAULT'); $fields_form[0]['form'] = array( 'legend' => array( 'title' => $this->l('Settings'), ), 'input' => array( array( 'type' => 'text', 'label' => $this->l('Steam key'), 'name' => 'steamkey', 'required' => true ) ), 'submit' => array( 'title' => $this->l('Save'), 'class' => 'button' ) ); $helper = new HelperForm(); $helper->module = $this; $helper->name_controller = $this->name; $helper->token = Tools::getAdminTokenLite('AdminModules'); $helper->currentIndex = AdminController::$currentIndex.'&configure='.$this->name; $helper->default_form_language = $default_lang; $helper->allow_employee_form_lang = $default_lang; $helper->title = $this->displayName; $helper->show_toolbar = true; $helper->toolbar_scroll = true; $helper->submit_action = 'submit'.$this->name; $helper->toolbar_btn = array( 'save' => array( 'desc' => $this->l('Save'), 'href' => AdminController::$currentIndex.'&configure='.$this->name.'&save'.$this->name. '&token='.Tools::getAdminTokenLite('AdminModules'), ), 'back' => array( 'href' => AdminController::$currentIndex.'&token='.Tools::getAdminTokenLite('AdminModules'), 'desc' => $this->l('Back to list') ) ); $helper->fields_value = array( 'steamkey' => Configuration::get($this->prefixed('steamkey')) ); return $helper->generateForm($fields_form); } public function prefixed($key) { return strtoupper($this->name.$key); } |

PrestaShop Support & Maintenance
Take your online store to the next level with BelVG PrestaShop Support & Maintenance
Visit the pageWe will also need to create a table to save SteamCommunity users data. For that let’s create the file /modules/steamlogin/sql_install.php and add the install() method to the main module file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<?php $sql = array(); $sql[] = 'CREATE TABLE IF NOT EXISTS `%1$s%2$s` ( `id_%2$s` int(10) UNSIGNED AUTO_INCREMENT, `steamid` varchar(255) NOT NULL, `id_customer` int(10) UNSIGNED NULL DEFAULT NULL, `personaname` varchar(255) NULL DEFAULT NULL, `realname` varchar(255) NULL DEFAULT NULL, `timecreated` datetime NULL DEFAULT NULL, `loccountrycode` varchar(2) NULL DEFAULT NULL, `locstatecode` varchar(5) NULL DEFAULT NULL, `loccityid` int(10) NULL DEFAULT NULL, CONSTRAINT `%1$s_pk_%2$s` PRIMARY KEY (`id_%2$s`), CONSTRAINT `%1$sfk_%2$s_customer` FOREIGN KEY (`id_customer`) REFERENCES `%1$scustomer` (`id_customer`) ON DELETE CASCADE ON UPDATE CASCADE )ENGINE=%3$s DEFAULT CHARSET=utf8;'; ?> |
Next, create a new SteamCustomer.php class and connect it to the main file.
The class SteamCustomer will contain such methods as: creating a new user, getting user’s ID by his Steam ID and vice versa.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 |
<?php class SteamCustomer extends ObjectModel { public $steamid; public $id_customer; public $personaname; public $realname; public $timecreated; public $loccountrycode; public $locstatecode; public $loccityid; public static $definition = array( 'table' => 'steamlogin', 'primary' => 'id_steamlogin', 'multilang' => false, 'fields' => array( 'steamid' => array('type' => self::TYPE_STRING, 'validate' => 'isString', 'required' => true), 'id_customer' => array('type' => self::TYPE_NOTHING, 'validate' => 'isUnsignedInt'), 'personaname' => array('type' => self::TYPE_STRING), 'realname' => array('type' => self::TYPE_STRING), 'timecreated' => array('type' => self::TYPE_STRING), 'loccountrycode' => array('type' => self::TYPE_STRING), 'locstatecode' => array('type' => self::TYPE_STRING), 'loccityid' => array('type' => self::TYPE_NOTHING) ) ); public static function createCustomer($steamid, $id_customer, $personaname, $realname, $timecreated, $loccountrycode, $locstatecode, $loccityid ) { $customer = new self(); $customer->steamid = $steamid; if ($id_customer) { $customer->id_customer = (int)$id_customer; } $customer->personaname = $personaname; if ($realname) { $customer->realname = $realname; } if ($timecreated) { $customer->timecreated = date('Y-m-d H:i:s', $timecreated); } if ($loccountrycode) { $customer->loccountrycode = (string)$loccountrycode; } if ($locstatecode) { $customer->locstatecode = (string)$locstatecode; } if ($loccityid) { $customer->loccityid = (int)$loccityid; } $customer->trade_url = ''; if ($customer->save(true)) { return $customer; } else { return new self(); } } public static function getSteamCustomerBySteamId($steam_id) { $steam_customer = new self(); $query = new DbQuery(); $query->from('steamlogin'); $query->where('steamid=\''.pSQL($steam_id).'\''); $result = Db::getInstance()->getRow($query); if ($result) { $steam_customer->arrayToObject($result); } return $steam_customer; } public static function getSteamCustomerByCustomerId($id_customer) { $steam_customer = new self(); $query = new DbQuery(); $query->from('steamlogin'); $query->where('id_customer='.(int)$id_customer); $result = Db::getInstance()->getRow($query); if ($result) { $steam_customer->arrayToObject($result); } return $steam_customer; } public function arrayToObject($array) { foreach ($array as $key => $value) { if (property_exists($this, $key)) { $this->{$key} = $value; } } $this->id = $array['id_steamlogin']; } } |
We will require the following hooks:
1 2 |
hookDisplayHeader($params); hookDisplayAdminCustomers($params); |
We will use hookDisplayAdminCustomers to output the info in the admin panel:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public function hookDisplayAdminCustomers($params) { $steam_customer = SteamCustomer::getSteamCustomerByCustomerId((int)Tools::getValue('id_customer')); if (Validate::isLoadedObject($steam_customer)) { $this->smarty->assign('steam_customer', $steam_customer); return $this->display(__FILE__, 'admin.tpl'); } } |
The template admin.tpl for the hook hookDisplayAdminCustomers contains the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
<div> <h2>{l s='Steam data' mod='steamlogin'}</h2> {l s='Steam id'}: {$steam_customer->steamid}<br/> {l s='Display name'}: {$steam_customer->personaname}</br> {l s='Real name'}: {$steam_customer->realname}<br/> {l s='Time created'}: {$steam_customer->timecreated}<br/> </div> |
Next, we will need to add the button that will let us authorize in SteamCommunity. For that we will use hookDisplayHeader.
1 2 3 4 5 6 7 |
public function hookDisplayHeader($params) { $this->hookFbLoginButton($params); } |
Next we verify whether a user profile is already present in the system and if not – generate an url in SteamCommunity.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
public function hookFbLoginButton($params) { if (!isset($this->context->customer->id) || empty($this->context->customer->id)) { $back = Tools::getShopProtocol()."{$_SERVER['HTTP_HOST']}{$_SERVER['REQUEST_URI']}"; if (!Tools::secureReferrer($back)) { $back = false; } $this->context->controller->addCSS($this->_path.'views/css/style.css'); $this->smarty->assign(array( 'steamlogin_url' => $this->context->link->getModuleLink($this->name, 'login', array('back' => $back)), 'img_path' => $this->_path.'views/img/', )); return $this->display(__FILE__, 'button.tpl'); } } |
The button template:
1 2 3 4 5 |
<form action="{$steamlogin_url|escape:'htmlall':'UTF-8'}" method="post" id="steam-login-form"> <input type="image" src="{$img_path}sits_small.png"/> </form> |
After the successful registration and in case of absense of such a user profile in Prestashop we are forwarded to the Prestashop registration form.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
<h1>{l s='Complete registration' mod='steamlogin'}</h1> <p class="required"><sup>*</sup>{l s='Required field' mod='steamlogin'}</p> {if isset($errors) && $errors} <div class="error"> <p>{if $errors|@count > 1}{l s='There are %d errors' sprintf=$errors|@count mod='steamlogin'}{else}{l s='There is %d error' sprintf=$errors|@count mod='steamlogin'}{/if}</p> <ol> {foreach from=$errors key=k item=error} <li>{$error}</li> {/foreach} </ol> </div> {/if} <form method="POST" action="{$steamlogin_url|escape:'htmlall':'UTF-8'}" class="std" id="steamform"> <fieldset class="steam_account_creation"> <p class="required text"> <label for="email">{l s='Email' mod='steamlogin'}<sup>*</sup></label> <input type="text" class="text" id="email" name="email" value="{$email}"> </p> <p class="required text"> <label for="firstname">{l s='First Name' mod='steamlogin'}<sup>*</sup></label> <input type="text" class="text" id="firstname" name="firstname" value="{$firstname}"> </p> <p class="required text"> <label for="lastname">{l s='Last Name' mod='steamlogin'}<sup>*</sup></label> <input type="text" class="text" id="lastname" name="lastname" value="{$lastname}"> </p> <p class="required text"> <label for="phone">{l s='Mobile Number' mod='steamlogin'}<sup>*</sup></label> <input type="text" class="text" id="phone" name="phone" value="{$phone}"> </p> <p class="required select"> <label for="country">{l s='Country' mod='steamlogin'}<sup>*</sup></label> <select name="country" id="select"> {foreach from=$countries item=country} <option value="{$country.id_country}">{$country.name}</option> {/foreach} </select> </p> <p class="required text"> <label for="date_of_birth">{l s='Date of Birth' mod='steamlogin'}<sup>*</sup></label> <input type="date" class="text" id="date_of_birth" name="date_of_birth" value="{$date_of_birth}"> </p> <p class="required text"> <label for="trade_url"><a href="#trade_url_header">{l s='Trade url (Steam)' mod='steamlogin'}</a><sup>*</sup></label> <input size="50" type="text" class="text" id="trade_url" name="trade_url" value="{$trade_url}"> </p> <p class="submit clearfix"> <input type="submit" name="submitAccount" id="submitSteamAccount" value="{l s='Save' mod='steamlogin'}" class="exclusive"> </p> <h2 id="trade_url_header">{l s='How to get Your Trade offer url:' mod='steamlogin'}</h2> <ul class="steam-list"> <li><a target="_blank" href="https://steamcommunity.com/my/tradeoffers/privacy#trade_offer_access_url">{l s='Click this Steam link >>>' mod='steamlogin'}</a></li> <li>{l s='Scroll down to "Third-Party Sites"' mod='steamlogin'}</li> <li>{l s='Copy "Trade URL"' mod='steamlogin'}</li> </ul> </fieldset> </form> |

PrestaShop Development
Take your online store to the next level with BelVG PrestaShop Development
Visit the page