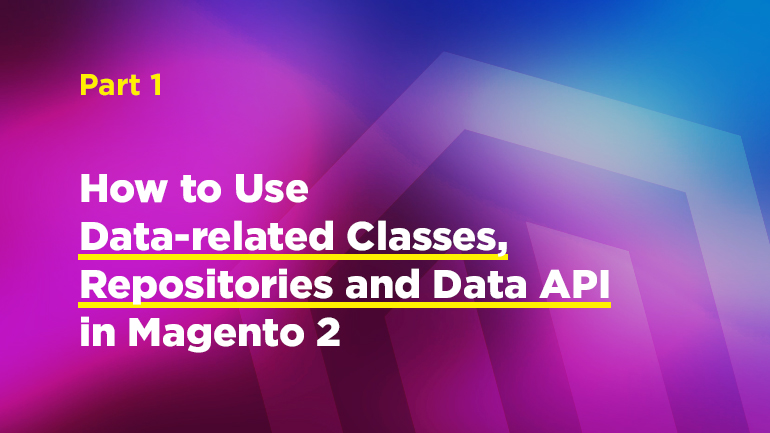
This is Part 1 of the series of articles dedicated to using data-related classes, repositories and data API in Magento 2. Today we will touch upon two questions: obtaining an object or set of objects from the database using a repository and configuring and creating a SearchCriteria instance using the builder.
Obtaining an object or set of objects from the database using a repository
To work with collections in Magento 2, you can use a repository that implements the same repository pattern. The Repository pattern allows working with collections as entities, regardless of their storage location (storage or caching are implementation details). The pattern itself is located between the Domain and the Service Level.
In Magento 2, there are usually 5 basic functions of the repository: save, getById, getList, delete, deleteById. But each Repository implementation in Magento has its own interface and these functions are not always implemented in it. For example, in Magento\Quote\Api\GuestCartTotalRepositoryInterface, only the get($cartId) method is implemented. Therefore, it is better to pay attention to the implementation of a particular Repository class.
Let’s take a look at the Repository using the example of \Magento\Catalog\Api\ProductRepositoryInterface and its implementation \Magento\Catalog\Model\ProductRepository.
1 2 3 4 5 6 7 8 9 10 11 |
<?php namespace Magento\Catalog\Api; interface ProductRepositoryInterface { public function save(\Magento\Catalog\Api\Data\ProductInterface $product, $saveOptions = false); public function get($sku, $editMode = false, $storeId = null, $forceReload = false); public function getById($productId, $editMode = false, $storeId = null, $forceReload = false); public function delete(\Magento\Catalog\Api\Data\ProductInterface $product); public function deleteById($sku); public function getList(\Magento\Framework\Api\SearchCriteriaInterface $searchCriteria); } |
Here all 5 basic functions for working with Repository are implemented. Meanwhile, the main class when working with a product is \Magento\Catalog\Api\Data\ProductInterface, which allows you to save all products inherited from this class, regardless of their type.
Let’s look at the implementation of the getById method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
<?php … protected $productRepository; public function __construct( … \Magento\Catalog\Api\ProductRepositoryInterface $productRepository) { $this->productRepository = $productRepository; } ... public function someMethod() { $product = $this->productRepository->getById(1); return $product->getSku(); } … ... public function someDeleteMethod() { $this->productRepository->deleteById(1); } … |
Here we implement the work with ProductRepository, extracting the object with id 1 and returning its SKU. Similarly, the deleteById method will be implemented. In the constructor, as you can see, we create \Magento\Catalog\Api\ProductRepositoryInterface, and not \Magento\Catalog\Model\ProductRepository itself. These dependencies are described in the di.xml file. For example, for the current class, the dependency is described in vendor/magento/module-catalog/etc/di.xml and looks like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<preference for="Magento\Catalog\Api\ProductRepositoryInterface" type="Magento\Catalog\Model\ProductRepository" /> Here is the implementation of the save method: ... public function someMethod() { $product = $this->productRepository->getById(1); $product->setSku(‘test-sku-1’); $this->productRepository->save($product); } … ... public function someDeleteMethod() { $product = $this->productRepository->getById(1); $this->productRepository->delete($product); } … |
Here we have changed the SKU of the product and saved it using the save repository method. The delete method works similarly (but with the opposite result).
Configuring and creating a SearchCriteria instance using the builder
The getList method, in this context, is implemented using SearchCriteriaInterface. This interface implements the conditions for queries (for example, in MySQL this is where in the query). All conditions are divided into Filter and FilterGroup.
We implement the abovementioned conditions using SearchCriteria:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
public function someMethod() { $filter = $this->objectManager->create('Magento\Framework\Api\Filter'); $filter->setField(‘sku’); $filter->setValue(‘test-%’); $filter->setConditionType(‘like’); $filter2 = $this->objectManager->create('Magento\Framework\Api\Filter'); $filter2->setField(‘sku’); $filter2->setValue(‘%-product’); $filter2->setConditionType(‘like’); $filterGroup = $this->objectManager->create('Magento\Framework\Api\Search\FilterGroup'); $filterGroup>setFilters([$filter, $filter2]); $filter3 = $this->objectManager->create('Magento\Framework\Api\Filter'); $filter3->setField(‘price’); $filter3->setValue(‘100’); $filter3->setConditionType(‘eq’); $filterGroup2 = $this->objectManager->create('Magento\Framework\Api\Search\FilterGroup'); $filterGroup2>setFilters([$filter3]); $searchCriteria- = $this->objectManager->create('Magento\Framework\Api\SearchCriteriaInterface'); $searchCriteria->setFilterGroups([$filterGroup, $filterGroup2]); $result = $this->productRepository>getList($searchCriteria-); return $result->getItems(); } |
This example returns an array of objects inherited from \Magento\Catalog\Api\Data\ProductInterface.
This is the end of part 1 of the series dedicated to data-related classes, repositories and data API in Magento 2. The other parts are coming up soon, stay tuned.

That was a good article
That was a good article