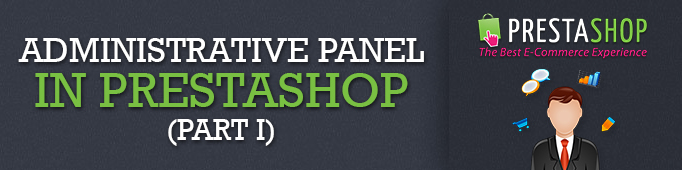
Administration panel is only used by store owners and staff. It’s not fancy-looking and it doesn’t have sliders, newsfeeds, blogs, social features or any other front-end eye-catchers. It’s very logical and extremely useful yet user-friendly. Administrators know it as a place that allows you to manage your system, edit products, manage your modules, view orders etc. How does it work?
Controllers
Controllers for the administration panel are located in this folder: site_path/controllers/admin/*.
They can also be placed in a module folder, for example: site_path/modules/belvg_stickerspro/AdminStickersPro.php
Controllers that are located in module folders are inherited from MоduleAdminCоntrоllerCоre, which, in turn, is inherited from the class AdminCоntrоllerCоre.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
abstract class ModuleAdminControllerCore extends AdminController { /** * @var Module */ public $module; public function __construct() { $this->controller_type = 'moduleadmin'; parent::__construct(); $tab = new Tab($this->id); if (!$tab->module) throw new PrestaShopException('Admin tab '.get_class($this).' is not a module tab'); $this->module = Module::getInstanceByName($tab->module); if (!$this->module->id) throw new PrestaShopException("Module {$tab->module} not found"); } public function createTemplate($tpl_name) { if (file_exists($this->getTemplatePath().$this->override_folder.$tpl_name) && $this->viewAccess()) return $this->context->smarty->createTemplate($this->getTemplatePath().$this->override_folder.$tpl_name, $this->context->smarty); return parent::createTemplate($tpl_name); } /** * Get path to back office templates for the module * * @return string */ public function getTemplatePath() { return _PS_MODULE_DIR_.$this->module->name.'/views/templates/admin/'; } } |
Let’s describe the functions of the class AdminCоntrоller:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 |
__construct() initBreadcrumbs() //Set breadcrumbs array for the controller page initToolbarTitle() //Set default toolbar_title to admin breadcrumb viewAccess($disable = false) //Check rights to view the current tab checkToken() //Check for security token ajaxProcessHelpAccess() //Use HelperHelpAccess processFilter() //Set the filters used for the list display Pоstprосess() //Process requests to the controller processDeleteImage() //Execute the function $object->deleteImage() of the current class processExport() //Export the function processDelete() //The function to delete the current class object processSave() //Call the right method for creating or updating object processAdd() //The function to add the current class object processUpdate() //The function to update the current class object processUpdateFields() //Change object required fields processStatus() //Change object status (active, inactive) processPosition() //Change object position processResetFilters() //Cancel all filters for this tab processUpdateOptions() //Update options and preferences initToolbar() //Assign default action in toolbar_btn smarty var, if they are not set. Override to specifically add, modify or remove items loadObject($opt = false) //Load class object using identifier in $_GET (if possible) otherwise return an empty object, or die checkAccess() //Check if the token is valid, else display a warning page filterToField() //Filter by field displayNoSmarty() //Does not have implementation displayAjax() //The function uses the layout-ajax.tpl template to display data redirect() // Header('Location: '.$this->redirect_after); Display() //Display content for the class $this- >layout displayWarning($msg) //Add a warning message to be displayed at the top of the page displayInformation($msg) //Add an info message to be displayed at the top of the page initHeader() //Assign smarty variables for the header addRowAction($action) //Declare an action to use for each row in the list addRowActionSkipList($action, $list) //Add an action to use for each row in the list initContent() //Assign smarty variables for all default views, list and form, then call other init functions initTabModuleList() //Init tab modules list and add button in toolbar addToolBarModulesListButton() //Add the button «Modules List» to the page with modules initCursedPage() //Initialize the invalid doom page of death initFooter() //Assign smarty variables for the footer renderModulesList() //Return $helper->renderModulesList($this->modules_list); renderList() //Function used to render the list to display for this controller renderView() //Override to render the view page renderForm() //Function used to render the form for this controller renderOptions() //Function used to render the options for this controller setHelperDisplay(Helper $helper) //This function sets various display option for a helper list setMedia() //This function connects css and js files l($string, $class = 'AdminTab', $addslashes = false, $htmlentities = true) //Non-static method which uses AdminController::translate() init() //Init context and dependencies, handles POST and GET initShopContext() //Init context for shop context initProcess() //Retrieve GET and POST value and translate them to actions getList($id_lang, $order_by = null, $order_way = null, $start = 0, $limit = null, $id_lang_shop = false) //Get the current objects' list form the database getModulesList($filter_modules_list) //Get the list of modules getLanguages() getFieldsValue($obj) //Return the list of fields value getFieldValue($obj, $key, $id_lang = null) //Return field value if possible (both classical and multilingual fields) validateRules($class_name = false) //Manage page display (form, list...) _childValidation() // Overload this method for custom checking viewDetails() // Display object details beforeDelete($object) // Called before deletion afterDelete($object, $oldId) //Called before deletion afterAdd($object) //The function is executed after it is added afterUpdate($object) //The function is executed after it is updated afterImageUpload() //Check rights to view the current tab copyFromPost(&$object, $table) //Copy datas from $_POST to object getSelectedAssoShop($table) //Returns an array with selected shops and type (group or boutique shop) updateAssoShop($id_object) //Update the associations of shops validateField($value, $field) //If necessary, the verifications goes via the Validate class beforeUpdateOptions() //The method, executed prior to options update function, does not have implementation by default postImage($id) //Overload this method for custom checking uploadImage($id, $name, $dir, $ext = false, $width = null, $height = null) //Image upload function processBulkDelete() //Delete multiple items processBulkEnableSelection() //Enable multiple items processBulkDisableSelection() //Disable multiple items processBulkStatusSelection($status) //Toggle status of multiple items processBulkAffectZone() //Execute the method affectZoneToSelection() of the current object beforeAdd($object) //Called before Add displayRequiredFields() //Prepare the view to display the required fields form createTemplate($tpl_name) //Create a template from the override file, else from the base file. jsonConfirmation($message) //Shortcut to set up a json success payload jsonError($message) //Shortcut to set up a json error payload isFresh($file, $timeout = 604800000) //Verify the necessity of updating cache refresh($file_to_refresh, $external_file) //Update the file $file_to_refresh with the new data from fillModuleData(&$module, $output_type = 'link', $back = null) //Fill the variables of the class Module displayModuleOptions($module, $output_type = 'link', $back = null) //Display modules list |
Let’s take a real constructor as an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
public function __construct() { $this->context = Context::getContext(); $this->table = 'belvg_stickerspro'; $this->identifier = 'id_belvg_stickerspro'; $this->className = 'Belvg_Stickerspro_Model'; $this->_defaultOrderBy = 'id_belvg_stickerspro'; $this->actions = array('edit', 'delete'); $this->bulk_actions = array('delete' => array('text' => $this->l('Delete selected'), 'confirm' => $this->l('Delete selected items?'))); //here it is determined which columns to output $this->fields_list = array( 'id_belvg_stickerspro' => array( 'title' => $this->l('ID'), 'width' => 25, 'align' => 'center', 'remove_onclick' => TRUE, ), 'image' => array( 'title' => $this->l('Photo'), 'align' => 'center', 'image' => 'p', 'width' => 70, 'orderby' => FALSE, 'filter' => FALSE, 'search' => FALSE), 'admin_name' => array( 'title' => $this->l('Sticker'), 'width' => 'auto', 'filter_key' => 'admin_name', 'align' => 'left', 'remove_onclick' => TRUE, )); /* $this->_join = ' LEFT JOIN `' . _DB_PREFIX_ . 'belvg_stickerspro_product` bsp ON (bsp.`id_belvg_stickerspro` = a.`id_belvg_stickerspro`) LEFT JOIN `' . _DB_PREFIX_ . 'product` p ON (p.`id_product` = bsp.`id_product`) LEFT JOIN `' . _DB_PREFIX_ . 'product_lang` pl ON (p.`id_product` = pl.`id_product` AND pl.`id_lang` = ' . (int) $this->context->language->id . ')'; $this->_select = 'p.active, pl.name '; $this->_group = ' GROUP BY bsp.id_product'; */ //you can change the request yourself parent::__construct(); } |

PrestaShop Modules
Take your online store to the next level with BelVG PrestaShop modules
Visit the storeLooking for expert PrestaShop development providers? Turn to BelVG!
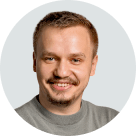
from where can i get class name please tell me how to define class name
Soufiane,
The route to override the controller template: override/controller/controllers/admin/templates. If you look at the function classes/controller/AdminController.php -> display() you will see that the path to the header.tpl file depends on the controller. So you can override it only for a particular controller but doe the whole site.
Hi,
Thank you for your tuto, but i didn’t work for me, i have prestashop 1.6.11 and i want to override header.tpl and footer.tpl of admin theme.
I puted them to override/controller/controllers/admin/templates
But nothing happined
Nadeem,
Please, check out this article:
http://blog.belvg.com/how-to-implement-a-controller.html
But in general, you should create a custom controller in the admin panel (it used be called Tab) and there you will be able to do whatever you like.
Thanks for the useful functions, I’ve a question:
how can I create a blank template to print orders (don’t need header footer, wanted to use my own smarty simple template to show specific information by using a template?
Thax
Karthik,
Basically, orders can be filtered out only by their status, which is assigned by the carrier. To filter by method of payment you need to customize the controller AdminOrders.
how to use the process filter function in order page to filter out only cash on delivery payment orders in backoffice order list page..
Chetаn,
Most likely you forgot to make Required for your custom Markers class within your AdminCоntrоller.
Hi
Can you please tell me I want to add class for the admin controller created by me but when I click on add new button it shows following error
Fatal error: Class ‘Makers’ not found in C:\wamp\www\prestashop\classes\controller\AdminController.php on line 1352
Kirti Umrigar ,
Please, check out how the function addRowAction of the class AdminController is implemented. To create the New button you need to call the function $this->addRowAction() in your AdminModuleController
Hello,
Nice Blog
Thank you
but can u tell me how to hide “Add New” and “Refresh List” button from the toolbar in listing page. (in back office)
Евгений,
You can verify your query by debugging this variable $this->_listsql in AdminController::getList()
I followed yours instructions and came across a problem.
When I try to use “$this->_join =” or “$this->_select” result in Helper List is empty.
P.S. SQL query is correct.